The Real Challenge of Big Dreams: Staying the Course
Dan Tulpa - July 9, 2024Building a Death Star isn't just about having the right tech or resources. It's the insane amount of time it takes.
Imagine starting a project that spans centuries. Society's mood swings could leave you with a galaxy full of half-built megastructures because halfway through, everyone decided it was a lame idea.
We live in an age where technological advances are skyrocketing. We’ve got materials that would blow your mind, energy sources that can power the unthinkable, and knowledge deeper than ever.
But what's rare?
The ability to stick with a big dream long enough to actually see it through.
Think about it.
Building a rocket that travels to the nearest star over 500 years is technically easier than making sure the crew’s grandkids keep that same fire and drive to continue the journey. Chances are, halfway there, they might just say, "Screw this," turn around, and head back to where things are safe and certain. They didn’t sign up for their ancestors’ wild dreams.
Now lets fast forward 250 years from launch.
Back home, there's bound to be flashy new tech, fresh ideas, maybe even new exploration methods that make the original mission look like a child’s drawing. Not to mention, the minds of those on board will evolve with the times too — what was mission-critical then could be irrelevant now.
An advanced civilization left to its own devices could, over millennia, craft AIs and tools to create virtually any material it dreams up, mastering the cosmos from the comfort of home. No scarcity of materials, energy, or knowledge.
The real scarcity?
A long-term attention span.
That’s not something you can just buy or download. We’d need groundbreaking ways to pass down values and missions across generations without turning them into burdens.
And here lies a DEEP ethical pickle.
How do you ethically pass down a mission centuries old without shackling future generations to the whims of their ancestors?
It’s a fine line between gifting opportunities and imposing outdated obligations. Odds are, future folks might see our grand plans as ancient missteps and choose paths we never even imagined.
Breaking the continuity of a long-term mission is easy when it stretches beyond a single lifetime, maybe even beyond the lifespan of entire societies. It turns out, maintaining focus over centuries or millennia might just be the most common struggle for advanced civilizations across the galaxy.
The likelihood of any civilization sticking to a thousand-year project?
Slim.
And keeping a project relevant for 500 years?
Even slimmer.
So, here I am... reflecting:
What would it take for me to dedicate myself to finishing a project started by generations before me?
What would make me stick it out, not bail halfway through?
That’s the million-dollar question.
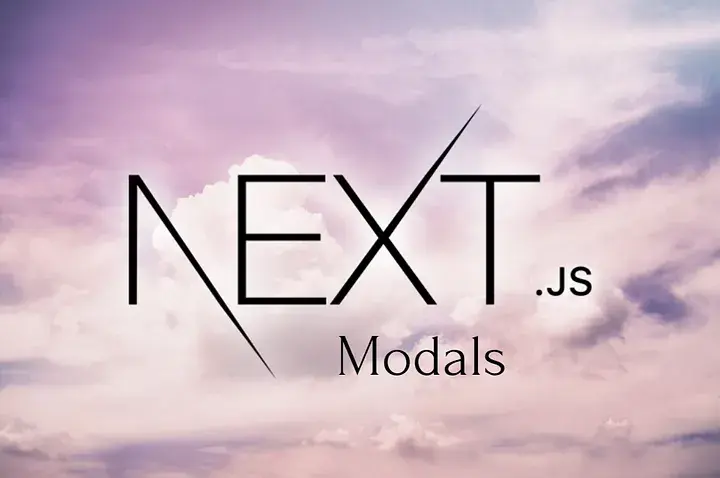
Next.js Server-Side Modals Made Easy
Dan Tulpa -Server-side Modals in Next.js: A Simplified Approach
Next.js is well-known for being strong and flexible, but sometimes its documentation, particularly when it comes to parallel and partial routes, can make simple things seem a bit complicated. This article aims to make the process of adding modals in Next.js easier to understand, keeping it simple and clear!
The Stateless, Route-Based Approach
The Concept:
- Stateless: This technique does not use useState or similar state management tools.
- Route-Based: It uses URL search parameters to control the modal’s visibility.
How It Works
Opening the Modal: The server component activates the modal when the URL includes the search parameter
?show=true
. More on this later!
Conditional Rendering: The page assesses the search parameters and then conditionally renders the Modal based on their presence.
Dismissing the Modal: To close the modal, users have options:
- Use the Link component to navigate back while maintaining the server-side nature.
- Utilize useRouter for a more dynamic, client-side approach.
Implementing the Modal 🔨
Here’s a server component example that conditionally renders a modal based on URL search parameters:
// page.tsx
import Link from "next/link";
import Modal from "@/components/Modal"
type SearchParamProps = {
searchParams: Record<string, string> | null | undefined;
};
export default function Page({ searchParams }: SearchParamProps) {
const show = searchParams?.show;
return (
<>
<Link href="/?show=true">
SUMMON THE MODAL
</Link>
{show && <Modal />}
</>
);
}
The above code is rendering in a <Link>
button with the text SUMMON THE MODAL
. There is also a Logical AND (&&) statement that is controlling when the <Modal/>
Component will be rendered.
When you click the button (our <Link>
tag), ?show=true
is added as a search parameter to the URL. This action triggers the conditional rendering of the modal, as the variable show becomes set to true.
Button Clicked
Modal Visibility 👀
Opening the Modal: The modal appears when the URL includes:
?show=true
.
Closing the Modal: To dismiss, users can either navigate back, use a specific close button, or remove the parameter from the URL.
Modal Component
Your modal component can be either a server component or a client component.
If you use Next’s <Link />
to dismiss the modal, it will remain a server component.
// Server-side Modal
function Modal() {
return (
<div className="fixed inset-0 bg-gray-600 bg-opacity-50 overflow-y-auto h-full w-full flex items-center justify-center">
<div className="p-8 border w-96 shadow-lg rounded-md bg-white">
<div className="text-center">
<h3 className="text-2xl font-bold text-gray-900">Modal Title</h3>
<div className="mt-2 px-7 py-3">
<p className="text-lg text-gray-500">Modal Body</p>
</div>
<div className="flex justify-center mt-4">
{/* Navigates back to the base URL - closing the modal */}
<Link
href="/"
className="px-4 py-2 bg-blue-500 text-white text-base font-medium rounded-md shadow-sm hover:bg-gray-400 focus:outline-none focus:ring-2 focus:ring-gray-300"
>
Close
</Link>
</div>
</div>
</div>
</div>
);
}
If you need more flexibility, you have to use useRouter and ditch the server component in favor of a client component. Only the client component can use useRouter.
//Client-side modal
'use client'
import { useRouter } from "next/router";
function Modal() {
const router = useRouter()
return (
<div className="fixed inset-0 bg-gray-600 bg-opacity-50 overflow-y-auto h-full w-full flex items-center justify-center">
<div className="p-8 border w-96 shadow-lg rounded-md bg-white">
<div className="text-center">
<h3 className="text-2xl font-bold text-gray-900">Modal Title</h3>
<div className="mt-2 px-7 py-3">
<p className="text-lg text-gray-500">Modal Body</p>
</div>
<div className="flex justify-center mt-4">
{/* Using useRouter to dismiss modal*/}
<button
onClick={router.back}
className="px-4 py-2 bg-blue-500 text-white text-base font-medium rounded-md shadow-sm hover:bg-gray-400 focus:outline-none focus:ring-2 focus:ring-gray-300"
>
Close
</button>
</div>
</div>
</div>
</div>
);
}
Advantages of This Approach ✅
- Simplicity: A simpler alternative to the more complex scenarios described in Next.js documentation.
- Stateless Design: Reduces complexity and boosts performance.
- Shareability: Enables direct link sharing to open modals.
- Performance: More efficient without the need for additional state management.
Conclusion
Implementing modals in Next.js doesn’t need to be overly complicated. By using a stateless, route-based approach, you can create efficient, user-friendly modals. This method is not only in line with modern web development practices but also simplifies the process, making it accessible for beginners and seasoned developers alike.
Thanks for reading, everyone. I hope this helps!
Keep hacking,
Dan
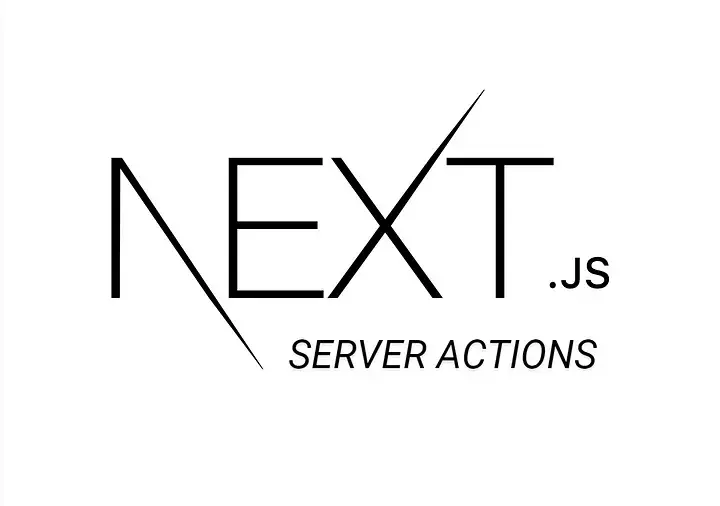
Next.js 14 Server Actions Tutorial
Dan Tulpa -Next 14 Server Actions Tutorial
In today’s article, we’re diving into the world of Next.js server actions to create a practical Todo application. Focusing on essential development practices, we’ll navigate through using the fetch() function, creating routes, and utilizing server actions in both Server and Client components.
By the end of this walkthrough, you’ll gain proficiency in server-side operations and client-side interactivity with Next.js, empowering you to build and manage dynamic web applications. Lets get started!
Getting Started
To create a Next.js app, open your terminal, cd into the directory you’d like to create the app in, and run the following command:
npx create-next-app@latest todo-app --example "https://github.com/dtulpa16/nextjs-todo-app"
Navigating the project
The codebase for this tutorial largely comes pre-written, simulating a real-world scenario where you often engage with established code. This setup is designed to direct your attention to mastering Server Actions, rather than building everything from scratch.
After installation, open the project in your code editor and navigate to nextjs-todo-app.
cd todo-app
Folder structure
You’ll notice that the project has the following folder structure:
app/
├── actions.ts
├── lib/
│ └── types.ts
├── api/
│ └── todos
│ └── route.ts
├── components/
│ ├── TodoFormCS.tsx
│ ├── TodoFormSS.tsx
│ └── TodoList.tsx
├── page.tsx
└── layout.tsx
public/
└── data.json
/app : Contains all the routes, components, and logic for your application, this is where you'll be mostly working from.
/app/actions.ts : Contains a server action to handle adding a new To-do to our mock database - more on this later!
/app/components : Contains all components we’ll be using to craft our UI
/app/api/todos/route.ts : Contains our API logic used to fetch the To-dos from our mock database
/public/data.json : Contains our To-Do data - will serve as our mock database.
Fetch API Data
We’ll be using our Next.js app as an API to fetch our To-Dos from our data.json file. Lets make fetch request to GET these To-Dos.
Task 1 - Finish the fetch() request in the app/components/ToDoList.tsx
to send a GET request to our local API and fetch our To-Dos
// app/components/ToDoList.tsx
const todos = await fetch("http://localhost:3000/api/todos", {
next: { tags: ["ToDo"] }
});
Notice we’re giving this fetch() request a tag - “ToDo”. We’ll be utilizing this tag later when we want to refetch our To-Dos after posting a new one to our database.
Your TodoList.tsx
should now look like this:
//app/components/ToDoList.tsx
import React from "react";
import { toDo } from "../lib/types";
export default async function ToDoList() {
// Fetch To-Dos from our route handler
const todos = await fetch("http://localhost:3000/todos/api", {
next: { tags: ["ToDo"] },
});
// Parse response to JSON
const { data } = await todos.json();
return (
<div className="max-w-xl mx-auto pt-10">
<h1 className="text-4xl font-bold mb-5">To-Do List</h1>
<ul>
{data.map((todo: toDo) => (
<li
key={todo.id}
className="bg-gray-800 p-4 rounded-lg mb-2 flex justify-between"
>
<div>{todo.task}</div>
<div className="bg-blue-600 px-2 py-1 rounded text-sm">
{todo.dueDate}
</div>
</li>
))}
</ul>
</div>
);
}
We want this list to render on our Homepage
Task 2 - Render this component in the app/page.tsx
//app/page.tsx
import ToDoList from './components/TodoList'
export default function Home() {
return (
<main>
<ToDoList/>
</main>
)
}
Task 3 - Run npm run dev
then navigate to http://localhost:3000 to see the list of To-dos fetched from our API — A Next.js Route Handler
Side quest — modify the styles of the due date
In ToDoList.tsx
:
Change <div className="bg-blue-600 ...">
to <div className="bg-green-500 ...">
Modify the text color by adding text-gray-900 to the same <div> tag
Adding our To-Do Form
Lets now get into using server actions to POST a new To-Do to our database!
Task 4 - Navigate to ToDoFormSS.tsx component, and you'll see that the form :
- Has two <input> elements for the task with name="task" and the due date name=”dueDate”
- Has one button with type="submit".
Task 5 - Create a folder named todo, nest a folder inside of that folder and call it create, then add a page.tsx to the create folder
└── app/
└── todo/
└── create/
└── page.tsx
This will create a new segment we can navigate to in our browser — http://localhost:3000/todo/create
Lets get some UI in our new route
Task 6 - Back in app/todo/create/page.tsx
, render in the ToDoFormSS.tsx
:
//app/todo/create/page.tsx
import TodoFormSS from '@/app/components/TodoFormSS'
export default function ToDoFormPage() {
return (
<TodoFormSS/>
)
}
Routing to our To-Do Form
Currently, the only way to view the UI for our To-Do form is to manually navigate to todo/create/ in our browser. Lets change that by adding a link on our Home Page that will route us to our new page
Task 7 - Add a navigation link to the app/page.tsx
that will route us to our new page :
//app/page.tsx
import Link from "next/link";
import ToDoList from "./components/TodoList";
export default function Home() {
return (
<main className="">
{/* Add A Link tag */}
<Link
href="/todo/create"
className="block mx-auto text-4xl font-bold mb-5 w-1/2 text-center pt-12 px-4 underline"
>
Add a To-Do
</Link>
<ToDoList/>
</main>
);
}
Task 8 - Save all, then navigate back to http://localhost:3000 in your browser and click on the link to be routed to the To-Do Form
Adding a Server Action — Post a To-Do
Now that we have our form created, lets add in our first server action to handle a user submitting a new To-Do
Within ToDoFormSS.tsx
, there's a function named addTodo()
that acts as our server-side hero. It's tagged with "use server" to ensure it doesn't run client-side, keeping our operations secure and efficient:
// app/components/ToDoFormSS.tsx
const addTodo = async (data: FormData) => {
"use server";
//...
}
- We extract the input values directly from the form data:
const task = data.get("task")?.toString();
const dueDate = data.get("dueDate")?.toString();
- With these values, we craft a new object to represent our to-do and shoot it over to our API using a POST request:
const newTodoBody = {
task: task,
dueDate: dueDate,
};
// Post new Todo to our mock database
await axios.post("http://localhost:3000/api/todos/", newTodoBody);
- After the new to-do is dispatched to our mock database, we use
revalidateTag("ToDo")
to fetch the updated catalog, including the new entry. Then, we navigate back to the home page
// Refetch Todo's
revalidateTag("ToDo");
// Redirect them back to the Homepage
redirect("/");
✍️ _Under the hood, we are using the FormData API here. This is a native browser API that allows the usage of forms to mutate data without the use of JavaScript. I highly recommend taking a look at the wealth of information available in the MDN docs!
All that’s left is to tie the addTodo
action to our form.
Task 9 - Add an action attribute pointing to addTodo
, and you're all set!
<form action={addTodo} className="space-y-4">
{/* form fields */}
</form>
Congratulations! You’ve successfully implemented your first Server Action. Here’s what you should expect if everything is working correctly:
- You should be redirected to the / route on submission.
- You should see the new To-do at the bottom of the list.
Refactor — Extracting a Server Action 🛠️
To simplify the TodoFormSS.tsx
component, let's organize our code and move the server action to a separate file.
In the actions.ts
file, you will find the same server action used in our TodoFormSS.tsx
component. We will be updating the <form> in app/components/TodoFormSS.tsx
to use this extracted function instead of declaring it directly in the component.
Task 10 - Navigate to app/actions.ts
. Notice here the file is tagged with 'use server'
at the very top level
💡 By adding the 'use server', you mark all the exported functions within the file as server functions. These server functions can then be imported into Client and Server components, making them extremely versatile.
// app/actions.ts
"use server";
import axios from "axios";
import { revalidateTag } from "next/cache";
import { redirect } from "next/navigation";
export const addTodo = async (data: FormData) => {
// Logic to mutate form data...
const task = data.get("task")?.toString();
const dueDate = data.get("dueDate")?.toString();
const newTodoBody = {
task: task,
dueDate: dueDate,
};
// Post new Todo to our mock database
await axios.post("http://localhost:3000/api/todos", newTodoBody);
// Refetch Todo's
revalidateTag("ToDo");
// Redirect them back to the Homepage
redirect("/");
};
Task 11 - Remove the addTodo()
function from the app/components/TodoFormSS.tsx
The next step will clear the error you get after deleting this.
Task 12 - Import the addTodo()
from the actions.ts file into the app/components/TodoFormSS.tsx
component.
Your TodoFormSS.tsx should now look like this :
// app/components/TodoFormSS.tsx
import { addTodo } from "../actions"; // Importing the addTodo function
export default function TodoFormSS() {
return (
<div className="flex justify-center items-center h-screen bg-gray-900">
<div className="max-w-xl mx-auto px-4 w-full">
<h1 className="text-4xl font-bold mb-5">Add A New To-Do</h1>
{/* Invoke the action using the "action" attribute */}
<form action={addTodo} className="space-y-4">
{/* Inputs & Button */}
</form>
</div>
</div>
);
}
Task 13 - Test by adding a new To-do!
Server Actions In Client Components
In a dynamic application, it’s common to combine server-side operations with client-side functionality such as state management and navigation.
To facilitate this within our Next.js application, we can import server-side functions into client components, maintaining a clean separation of concerns.
Task 14 - We are now going to be rendering in our ToDoFormCS.tsx
component inside of our app/todo/create/page.tsx
Navigate to the TodoFormCS.tsx component, and you'll see:
- “use client” at the very top — marking this as a Client component
- Our addTodo function being imported
- Two state variables — task & dueDate
- A handleSubmit function
- A <form> with an action attribute set equal to the handleSubmit
- 2 <input> tags with onChange events that capture the user’s input in our state variables
We’re going to be replacing the Server-side To-do Form in our todo/create/ route with this client-side To-do Form
Task 15 - In app/todo/create/page.tsx
, replace <ToDoFormSS/>
with <ToDoFormCS/>
:
//app/todo/create/page.tsx
import TodoFormCS from '@/app/components/TodoFormCS'
export default function ToDoFormPage() {
return (
<TodoFormCS/>
)
}
Task 16 - Navigate to the http://localhost:3000/todo/create in your browser and you should see the same UI being rendered
Our Client side “Create Page” that utilizes Server Actions for handling POSTing data
Back in your TodoFormCS.tsx
component — in the handleSubmit(), you’ll see:
-
A new FormData instance being created key/values being added/appended to the form data instance
-
The addTodo() server action being called with the form data being passed in.
const handleSubmit = (event: any) => {
const formData = new FormData()
formData.append("task", task)
formData.append("dueDate", dueDate)
addTodo(formData)
};
In this function, we are replicating what happened in our server component when we submitted the form.
Our server function is expecting a FormData instance with values (our inputs) being a part of the form data.
Task 17 - Test it out by adding a new To-do, if everything is working correctly :
- You should be redirected to the / route on submission.
- You should see the new To-do at the bottom of the list.
Congrats! You’ve just implemented a server action in both a Server and Client side component!
Conclusion
Server Functions are functions that run on the server but can be called from the client, enhancing Next.js’s server-side abilities.
Server Actions are like Server Functions, but they’re activated by specific actions. By connecting with form elements, particularly via the action prop, they keep forms interactive even before client-side JavaScript is loaded. This means users can submit forms smoothly without waiting for React hydration.
That's a wrap for this tutorial! Thanks for sticking around. Hope you all learned something!
Keep hacking,
Dan
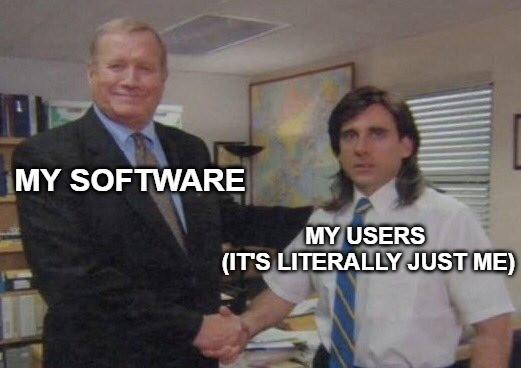
Why I Build Apps That Nobody Uses
Dan Tulpa -Hey everyone, or maybe just me and my girlfriend if you're the only ones reading this. In which case, hi Megan!!
So, a bit about me:
I build apps.
A LOT of apps.
Well over 15 passion projects to date, to be exact.
In my eyes, they’re unique and full of character. I’m talking about apps that do everything from generating custom healthy recipes based on insanely specific user inputs to simulating entire job interviews complete with AI driven feedback (P.S. I'm talking about this app here 😉).
I like to use the OpenAI API to do snazzy things like stream AI responses in Slack (COMPLETELY unnecessary, but damn do I think it’s cool) - stuff I hadn’t seen anyone else do when I first started messing around with it.
And don’t even get me started on hosting everything. Backends, databases, and frontends spread across AWS, Azure, Netlify, and Heroku. Honestly I find that each service has its own standout feature that I like to see evolve, and its fun keeping up with each one’s updates.
Yes, it costs me about a hundred bucks a month, and yes, I pay for it out of my own pocket.
Why?
Because 👏 it's 👏 my 👏 passion 👏
I’ve planned, built, hosted, and styled all these projects myself, relying on nothing but good ol’ Tailwind CSS.
And despite the fact that these apps don’t really draw a crowd, I'm totally okay with that.
So, Why Do I Keep Building?
You’ve heard it before - “It's the journey; not the destination”.
I build because I love the process.
There’s just something special about bringing an idea to life, about dabbling with a new technology and bending it to your will. Each project is a learning experience, a creative outlet, a challenge that I set for myself.
The late nights troubleshooting a bug, the breakthrough moments when a piece of code FINALLY works as expected, and the “jump out of your seat” type of joy of seeing something I imagined come to life. You just can't beat that feeling.
I can’t lie, sometimes I wish more people - especially recruiters (thanks ATS) - could see what I’ve made. But at the end of the day, I’m doing this for me. I’m pushing my own boundaries, making “something out of nothing” , and having a blast while I’m at it.
Whats Next For Me
I’ll keep creating, keep learning, and who knows? Maybe one of these days, one of my projects will catch the right eye.
Until then, you can bet your bottom dollar that I’ll be here, coding away, building something amazing.
Each new project is an opportunity to try something different, to experiment with new technologies or to refine techniques I've already learned. It's this constant cycle of innovation and self-improvement that keeps me hooked.
And to anyone who has ever used one of my apps - even just once - it makes me very happy to know that my platforms aren't just floating out there in the digital ether. Your interest is truly what fuels my drive to keep innovating and creating.
For anyone out there who might be reading this, remember: find what you love, pour your heart into it, and don't stop. That's the spirit of a true creator.
Thanks for sticking around.
Keep hacking,
Dan
Drop the Ego: Why Asking for Help Makes You a Stronger Developer
Dan Tulpa -The Real Strength of a Developer: Knowing When to Ask for Help
Hey everyone, Dan here.
Today, we're tackling a topic close to my heart — the misconception that seeking help is a sign of weakness. Let's break that myth and explore why admitting defeat and asking for assistance is actually one of the smartest moves you can make in your tech career.
Strength in Community
During my three years as an instructor at a coding bootcamp, mentoring over a thousand students, I saw firsthand that coding isn’t a solitary pursuit. It's a team sport. The students who asked for help consistently outperformed those who didn’t. Period. We used to call it “struggling in silence” when someone wouldn’t ask for help, and it was always disheartening to see someone struggle for days on something that could have been resolved in minutes.
The Heavy Cost of Going In Alone
It's easy to fall into the trap of thinking you have to solve every problem on your own. This mindset, however, comes with significant drawbacks:
-
Stagnation: Have you ever found yourself stuck on a problem, spinning your wheels? That's what happens when pride dictates your actions. Avoiding help keeps you stuck and halts your progress.
-
Isolation: Flying solo might seem appealing, but it can lead to missing out on valuable connections and insights from others in the field.
-
Errors Galore: Tackling everything alone sets you up for a rough ride. Many mistakes could be easily avoided by simply reaching out.
Here’s what you gain by embracing the power of asking:
-
Accelerated Learning: Engaging with more experienced developers, asking questions, and overcoming obstacles quickly enhances your growth. It's about improving, not just scraping by.
-
Community Power: Being active in developer forums or local meetups connects you with a support network that is incredibly valuable.
-
Creative Solutions: Collaboration often leads to discovering solutions you might never have thought of on your own.
How to Embrace Asking for Help
Ready to let go of the 'lone wolf' act? Good! Here’s how to start:
-
Adopt a Growth Mindset: Believe that improvement is always possible. Focus on learning and growing, rather than just proving what you know.
-
Normalize Asking for Help: Start with small requests and gradually take on more. Turning asking into a habit removes the fear associated with it.
-
Seek Out Mentors: Find people who have been where you want to go. Their guidance can be invaluable, and their insights can accelerate your progress.
-
Engage Actively in Communities: Dive into the developer scene. It’s filled with opportunities to learn, share knowledge, and even make some friends.
-
Celebrate Your Wins: Reflect on the times when asking for help has led to success. Let these victories remind you of the effectiveness of reaching out.
Conclusion
Being an outstanding developer isn’t about doing it alone; it's about knowing when to ask for help and having the courage to do so. Drop the ego, embrace collaboration, and watch your growth accelerate.
Remember, the smartest people are the ones who know they don’t know everything.
Keep hacking,
Dan
Focus to Dominate: Why Junior Developers Should Master One Language First
Dan Tulpa -If you're just kicking off your dev career and you’re bombarded with advice to learn a dozen languages and frameworks to stay relevant — pump the brakes. Here’s the straight truth: focusing deeply on one language or technology before spreading yourself too thin will make you a better developer. Simple as that. This isn’t about taking it easy; it's about avoiding mediocrity. Let's break down why mastering one tech game first builds a rock-solid foundation and primes you for faster learning in the future.
Why Mastery Beats Dabbling: A No-BS Guide
Forge a Bulletproof Foundation
When you channel your energy into one language, you're not just learning to code; you're learning to think like a programmer. This deep dive means you grasp core concepts that cut across all tech languages. Get this right, and you’ve equipped yourself with a toolkit to tackle any programming challenge, regardless of the language.
Stand Out in the Job Hunt
Companies crave specialists, not generalists. Lock down expertise in one key area, and you’re the go-to person for that tech. That’s how you land top jobs and command higher salaries. Specialize first, and doors will open.
Speed and Efficiency
Trying to juggle multiple languages from the start is like spinning plates while learning to juggle — something's gonna crash. Focus sharpens your learning curve, skyrocketing your efficiency and proficiency. That’s how you fast-track your projects and career.
Boost Your Confidence
Nothing boosts confidence more than achieving success and overcoming challenging issues. When you become fluent in one language, your confidence will rise. Being a significant contributor to tech conversations and projects is the goal here, not merely feeling good.
Turning One Language Mastery into Many
It is surprisingly simple to pick up another language once you have mastered one. The abilities you have acquired are transferable. Crushing it with JavaScript? Great, you’ve got a handle on variables, loops, data structures, and more. Jump to another language, and it’s just a new syntax; the fundamentals are the same.
Skills That Transfer:
- Problem-solving: Good at untangling problems in one language? You’ll untangle them in any language.
- Debugging: Debugging isn’t language-specific. Learn to squash bugs in one, and you’ll squash them in all.
- Frameworks and Libraries: Get the hang of one set of tools, and you’ll understand how to approach any set.
How to Start Focusing Right Now
- Pick What Fires You Up: Choose a language that lines up with what you're passionate about—be it web development, mobile apps, or data science.
- Go All In: Dive deep. Build projects, get involved in the community, contribute to open source, and tackle coding challenges.
- Smart Resource Selection: Opt for quality learning resources. Don’t skim the surface with a scattergun approach—choose depth over breadth.
Wrap-Up
For the rookie devs out there, remember this: depth wins over breadth every time. A focused mastery of one programming language sets you up not just for immediate wins but for long-term career success. Don't just learn to code — learn to excel. This is how you make your mark in software development.
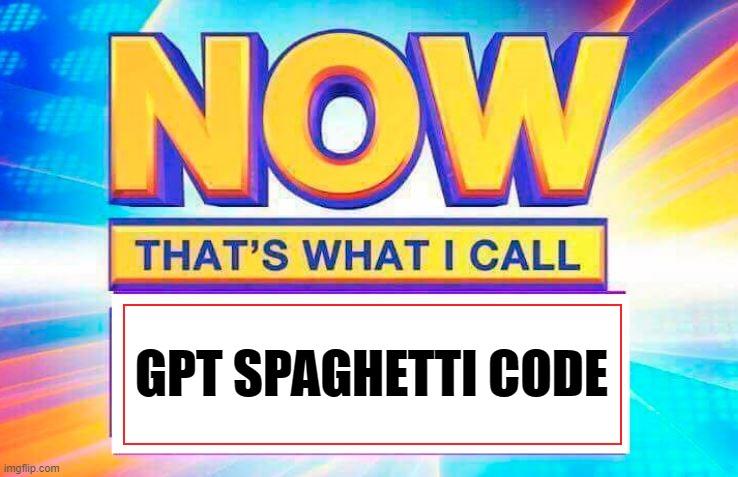
AI: The Tough Love Mentor for Junior Developers
Dan Tulpa -Let’s get straight to the point about artificial intelligence for junior developers. AI can be a remarkable tool, propelling your growth as a developer faster than you might think. However, if misused, it could potentially derail your career before it even starts.
Accelerating Development
The benefits are clear: AI tools act like an all-knowing mentor available around the clock. They can critique your code, identify inefficiencies, and even fix bugs in real time. This can significantly accelerate your learning curve, allowing you to enhance your skills quickly.
Moreover, AI can handle the repetitive and mundane tasks, freeing you up to focus on more complex and creative challenges. This not only boosts your job satisfaction but also keeps you engaged.
Risks of Overreliance
Depending too much on AI is like using training wheels—they help you ride, but don’t teach you balance. If AI does all the hard work, you miss out on the hands-on experience needed to develop your instincts and problem-solving abilities.
Overreliance on AI can stunt your growth, leaving you unprepared when facing problems that AI tools can’t solve. The goal should be to learn problem-solving, not just to get problems solved.
Striking the Right Balance
So, what’s the strategy? Use AI as a sparring partner, not a crutch. Junior developers should engage with AI in a way that enhances their abilities without overshadowing the essential skills they need to build.
Mentorship is crucial here. Experienced developers should guide juniors, ensuring that while AI handles the tedious tasks, juniors still tackle significant challenges. This approach prepares them for tougher problems and helps them become well-rounded, competent coders.
Wrapping Up
In essence, AI can significantly boost the careers of junior developers, accelerating their effectiveness at an unprecedented rate. However, without the right balance, they risk becoming mere operators rather than masters of their tools. As we integrate AI into development practices, we must ensure it enhances the developer experience rather than overtaking it.
Small Businesses vs. The Big Guys: Winning with AI
Dan Tulpa -Ever noticed how the main streets are changing? More chains, fewer local spots — it’s a shift you can't ignore.
Walking through my hometown, the slow takeover by the big guns is, honestly... very stark.
The real question is, how can the little guys punch above their weight, especially using AI, without breaking the bank?
Well, here’s the lowdown on making AI work hard for your small business in 2024.
1. Kick Ass with Customer Personalization
What’s the ace up your sleeve as a small business?
That personal touch.
AI cranks this up by getting smart with customer data.
Imagine offering tailor-made experiences that rival Amazon’s, without their kind of cash. Tools like Clerk.io and Klevu help personalize everything from recommendations to discounts, making your customers feel like VIPs.
2. Streamline Operations Like a Boss
Big corporations have efficiency down to an art, but AI’s here to even the playing field.
Think about it: A single AI-focused platform can handle your scheduling and inventory. Tools like Deputy and When I Work keep things slick and save you money, letting you focus on expanding your empire and keeping customers happy.
3. AI-Driven Customer Support
Great customer service is non-negotiable.
With AI chatbots, you’re never off the clock.
Platforms like Chatfuel and ManyChat hook right into your current systems, handling the usual queries so you can step in when it’s time to add the human touch.
4. Dominate Local Marketing with AI Insights
Understanding your turf is crucial, and AI is like having a local market guru on tap.
Use AI-enhanced tools like Google Analytics to spot trends and consumer behaviors that you’d otherwise miss.
It’s about targeting your marketing efforts to hit where it counts.
5. Boost Your Online Game
For many small businesses, the internet is your main street.
Tools like Wix and Squarespace aren’t just about building sleek websites.
They offer the muscle of AI to significantly improve your site’s analytics, SEO, and customer interactions, making sure you stand out in the digital crowd.
6. Smarten Up Your Social Media
Social media isn’t just about posting what and when you feel like.
AI tools like Buffer and Hootsuite help pinpoint the right content and timing for max engagement, keeping your feeds buzzing and your audience hooked.
Conclusion
The local business scene is definitely transforming, but AI gives you a fighting chance to not just survive but thrive.
It’s not about duking it out with giants like Walmart or McDonald’s - it’s about showcasing what makes you unique and fuel those qualities with sharp, accessible tech.
For small business owners, it's time to gear up: Harness AI, boost your inherent strengths, and cement your place in the community. Don’t count out the local champs yet; with AI, they’re smarter, tougher, and ready to take on the giants head-on.
AI and Human Evolution
Dan Tulpa -Ever feel like we're just skimming through life, barely scratching the surface of our potential? Enter artificial intelligence — not the emotionless, world-dominating entity pop culture makes it out to be, but a tool that could reshape our very existence.
Forget about AI fears for a moment and consider this: AI could be the key to unlocking human capabilities we’ve barely begun to tap into.
Cutting Through the AI Hype
Movies like "The Matrix" paint AI as humanity’s nemesis. But let's get real here. AI isn’t plotting our downfall. It doesn’t want anything. It's a tool, absent of desires or emotions, processing data and identifying patterns. Thats literally it...
I don't know about you, but I believe it’s time we move past these exaggerated sci-fi scenarios and recognize AI for what it truly is — a powerful asset empty of sentient motives.
AI as Your Personal Efficiency Hack
Tired of the daily grind? Overwhelmed by tasks that feel like they’re just eating away at your day? That’s where AI steps in. Let it handle the mind-numbing, repetitive tasks. Why? So you can focus on what fires you up! Whether that’s innovating in your field, crafting something new, or just finding better ways to solve big problems, AI is here to clear the mundane off your plate and ramp up your productivity.
Imagine diving deep into creativity without the constant nag of routine tasks. AI’s got your back, handling the dull stuff so you can play in the realms of imagination and impact.
Life Beyond the 9-to-5
With AI taking on the routine, we’re talking about more than just saving a few hours. We’re talking about a fundamental shift in how we live our lives. This isn’t about working less, it’s about living more .
Take a second to REALLY think about this - What passions would you explore if time wasn’t a constraint? Whether it's learning a new language, mastering the bagpipes, write your novel. AI can make that time—your most valuable resource—abundant.
Rekindling Human Connections
In the rush of our daily routines, our relationships often take a back seat.
But what if AI could help us change that?
By offloading our workloads, AI grants us something priceless — time for the people who matter most.
Imagine deeper conversations, more meaningful interactions, and stronger bonds — all because you aren’t just squeezing in time between endless tasks.
Rediscovering Your Purpose
Lost in the noise of daily obligations, it’s easy to drift away from our deeper goals.
AI offers a chance to step back and think — what really drives you? With more room for introspection, you can align closer with your passions and redefine your purpose.
It’s about making room for growth, reflection, and a deeper understanding of what makes you tick.
AI: The Catalyst for Human Evolution
Ready to shift how you see AI and its role in our future? It’s not about handing over control.. it’s about developing what makes us uniquely human. Our creativity, empathy, and ingenuity can all scale up with AI as our partner.
It's about harnessing technology to enhance our lives, not overshadow them.
Conclusion
It’s high time we shift the narrative around AI from fear to opportunity.
Let's embrace AI as a catalyst for evolution — freeing us from drudgery and opening up avenues for creativity, connection, and personal fulfillment.
Can you envision a future where technology and humanity thrive together?
That’s the journey we’re on.
With AI as a tool, not a tyrant, we can redefine the essence of what it means to live and excel. Take the reins and steer this ship towards a future where we all thrive—powered by AI but led by human spirit.
"Team Human" Decoded: A Real Talk on Humanizing Technology
Dan Tulpa -Douglas Rushkoff's "Team Human" hits like a wake-up call in our tech-saturated lives.
It's a battle cry for all of us drowning in digital noise, pushing us to reconnect with what's real—people.
This post dives into how "Team Human" challenges the tech world to bring back empathy, community, and some damn humanity to the workplace.
Straight Talk from "Team Human" and Its Knock-On Effects in Tech
Pump Up the Human Connection
Rushkoff is all about boosting real human interactions, especially in tech spaces where digital often drowns out the personal.
He’s pushing for a workplace that values face-to-face teamwork over isolated screen time.
Why?
Because it amps up creativity, employee happiness, and builds a kick-ass company culture.
Automate with Caution
"Team Human" throws a red flag on the mad rush towards automation.
Sure, it's slick, but at what cost?
Rushkoff challenges tech heads to think hard: Are we programming ourselves out of our own jobs?
The goal should be tech that complements human smarts, not tech that makes us redundant.
Cultivate Collaborative Zones
Rushkoff’s big on pulling people together to fight the cold tech tide.
He talks about crafting spaces where collaboration isn’t just a buzzword but the way we roll — where every idea is valued and respect runs deep.
It’s really about sparking innovation and building a place where everyone wants to work.
Ethics Aren’t Optional
Diving into the murkier waters, "Team Human" spotlights the ethical side of our digital doings.
This is a call to arms for tech pros to lead with integrity, ensuring privacy, societal perks, and thinking long-term about the impact of what they create.
We need to steer tech towards being a force for good, not just a money-maker.
Humans First, Technology Second
At its heart, Rushkoff’s message is clear: Value human experience over machine efficiency.
In the tech scene, this means designing products that enhance life and strengthen human bonds.
Technology should serve us, not the other way around.
Practical Ways to Bring "Team Human" Into Tech Workplaces
-
Boost Team Collaboration: Foster open communication and teamwork where everyone’s take is valued.
-
Lead with Empathy in Design: Innovate with a focus on truly enriching the user's life.
-
Stick to Ethical Standards: Weave ethical considerations into all stages of tech development, from concept to rollout.
-
Push for Diversity and Inclusion: Embrace diverse perspectives to fuel more innovative, human-centered technology.
-
Promote Digital Breaks: Encourage a healthy balance between screen time and offline activities to spark creativity and reduce burnout.
Conclusion
Douglas Rushkoff’s "Team Human" is a crucial manifesto for anyone in tech today.
It’s about more than just making a living; it’s about making a meaningful life in the digital age.
For tech folks ready to cut through the BS and build a future that values real connections and ethical action, "Team Human" is your handbook.
Let’s get to work and make tech about people again.
If You're Reading This, You Should Take A Screen Break
Dan Tulpa -Ever caught yourself zoned out, eyes burning, while wrestling with a relentless code bug?
You are not alone.
It's a typical moment for us developers, getting stuck between hammering away and knowing when to step back.
Here’s the raw truth: Taking regular breaks isn’t just about giving your eyes a rest—it's a badass strategy for sharper productivity and sanity.
The Undeniable Need for Screen Breaks
Amp Up Your Productivity
Sounds like a paradox, right?
Pause working to get more done?
Absolutely!
Our brains aren’t wired to grind non-stop.
Research shows short breaks keep your performance steady across the board, while plowing through can tank it.
For devs, stepping back means you might crack that code quicker than if you just brute-forced it.
Slash Eye Strain
Glaring at your screen too much?
Welcome to Computer Vision Syndrome—headaches, blurry vision, and dry eyes.
But there's a simple hack: the 20-20-20 rule.
Every 20 minutes, shift your gaze to something 20 feet away for 20 seconds.
It’s a small move with big benefits, helping dodge those nasty headaches.
Supercharged Problem-Solving
Ever stumbled upon a solution while grabbing a coffee or hitting the shower?
That’s your brain sorting shit out when you're not sweating the cursor.
Distance can decode complexities, letting your subconscious tackle them without pressure.
How to Nail Your Screen Break Game
Timed Breaks
Utilize tools like Pomodoro timers.
Work solid for 25 minutes; break for 5.
After four rounds, chill a bit longer, like 15 to 30 minutes.
It’s about keeping your brain’s attention sharp and your productivity smoking.
Get Physical. Serously.
Use your breaks to move.
Stretch, stroll, or just stand up. It pumps blood to your brain, refreshing your mental and physical state, so you can attack those coding problems with a vengeance.
Mind Over Matter
Try some mindfulness or breathing exercises while you're off-screen.
These are power tools for stress reduction and mental clarity, setting you up perfectly to tackle whatever’s next.
Real Talk: The Impact of Taking Breaks
From personal trenches in the coding world, I can tell you with confidence — regular screen breaks refine your focus and ramp up efficiency.
They transform coding marathons from mind-numbing spans to something you can actually enjoy.
More importantly, they keep your work-life balance in check, preventing you from burning out in the mad dash of constant deadlines.
So, next time you're knee-deep in code, remember that hitting pause is often the smartest move.
It’s not about slacking off — it's about hustling smart.
Integrity: The Unsung Hero in Software Development
Dan Tulpa -In the cutthroat world of software development, it's easy to get lost in the whirlwind of coding practices, emerging technologies, and relentless deadlines.
But let’s cut the crap — what really makes or breaks lasting success in this game?
Integrity.
Not just a high-minded concept, but a hardcore principle driving innovation, building trust, and ensuring sustainable success in tech.
Why Integrity is Your Best Tool in Software Development
Integrity is a rock-solid commitment to ethical coding, quality craftsmanship, owning your screw-ups, and learning from them.
Integrity is what turns a good developer into a great one.
Ethical Coding: Handle With Care
Think about it.
Every line of code you write, every algorithm you design has the power to affect lives.
Keeping your coding ethical means prioritizing user privacy and security like they were your own.
It’s about coding like someone’s life depends on it — because often, it does.
Quality Over Quick Fixes
Deadlines are breathing down your neck. The temptation to cut corners is real. But integrity means sticking to your guns and upholding quality.
Whether it’s a line of code, a user interface, or a software update, making sure it’s top-notch is non-negotiable. Quality isn’t just a standard; it’s a statement.
Own Your Mistakes
Messed up?
Who cares? You're a human, and that's just what humans do. Own it.
In software development, errors are as natural as breathing. What matters is stepping up, admitting the mess, and fixing it—no excuses.
This transparency earns you the trust and respect of your team and your users.
Fail Forward
Innovation isn’t all sunshine and rainbows. It comes with its fair share of faceplants.
Integrity means embracing these failures, learning the hard lessons, and using them to beef up your skills.
It’s about failing forward and turning screw-ups into step-ups.
The Real-World Impact of Integrity in Development
This isn’t about individual glory. The ripple effects of integrity in software development stretch far beyond any single project or developer.
Earn That Trust
Software’s got a role in nearly every aspect of life. When you stick to integrity, you’re not just churning out code — you’re building trust.
Users need to know that the software they depend on isn’t just functional but is also safe and respects their privacy.
Forge Stronger Teams
Integrity is the glue in killer teams. It creates an environment where open communication, mutual respect, and collaboration aren’t just encouraged; they’re expected.
It’s all about pushing everyone to bring their A-game, every time.
Drive Innovation
When your culture is steeped in integrity, you’re free to experiment and take risks—safely. Push boundaries and exploring new frontiers in technology without being a jerk about it.
Secure Your Future
Sure, doing things right might slow you down now, but it sets you up for lasting success.
Ethical practices and commitment to quality mean building software solutions that don’t just work today but keep on kicking ass into the future.
Bottom Line
In a world that moves as fast as the tech industry, integrity is your anchor. It’s what ensures that our digital universe rests on a foundation of trust, quality, and responsibility.
So, as we keep pushing the envelope on what software can do, let’s make sure we’re doing it with integrity — not just because it sounds good, but because it is good—for everyone.
Prioritizing Mental Health While Working Remotely
Dan Tulpa -Gone are the days of mind-numbing commutes and fluorescent-lit cubicles. Remote work has stormed the scene, flipping the traditional work environment on its head with its flexibility and no-commute lifestyle. But let's not kid ourselves; while the perks are real, so are the mental health battles that come tucked away in its pockets. This post is a hard look at the mental cost of remote work and how to fight back.
The Real Cost of Remote Work on Your Mental Game
Sure, working from your couch might sound like the dream setup, but it's not all sunshine and pajamas. The lines between home and work blur into a mess that can jack up stress, crank up isolation feelings, and push you toward burnout. Think I'm exaggerating? A survey by the American Psychological Association flags that nearly 60% of remote workers are wrestling with ramped-up work-related stress. And if that's not enough, one in three feel the sting of loneliness from missing out on real human connection.
Spotting the Warning Signs
Let's break it down; here's what to watch for:
- Sleep Troubles: Tossing all night or sleeping like it's your job? Both can be your mind's SOS signal.
- Slumping Productivity: If getting things done feels like moving mountains, you might be staring down burnout or anxiety.
- The Loneliness Factor: Feeling cut off from your team? It’s more than just missing water cooler chats—it can spiral into real-deal depression.
- Anxiety or Irritability: Overwhelmed by the small stuff? It could be your early warning system kicking in.
Battle Strategies for Your Mental Health in Remote Work
Carve Out a Routine
Command your day like a boss—set firm work hours, punctuate them with breaks, and shut it down when it's quitting time. Structure is your ally here; it keeps work from seeping into your downtime.
Set Up Your Battle Station
Create a space just for work. It’s about tricking your brain into flipping the 'on' switch for work mode and hitting 'off' when it’s time to chill.
Keep the Lines Open
Stay plugged into your network. Regular video calls, instant messaging, and virtual meets aren't just for updates—they're lifelines that tether you to the crew, fighting off the isolation monster.
Don't Skimp on the Physical
Your body's health is your mind’s armor. Hit the pavement, eat right, and catch those Zs. Regular workouts? Non-negotiable—they slash stress and beat back the blues.
Call in the Pros
No shame in the therapy game. If the mental weight feels too heavy, reach out for professional help. Remote doesn't mean out of reach—many therapists are just a call away.
Master Your Mind
Dive into mindfulness, meditation, or yoga. These aren’t just trendy; they’re tools to dial down stress and boost your mental state.
Wrapping Up
Remote work isn’t the villain here, but it’s not a free pass either. Keep your eyes open for the mental traps it sets. Arm yourself with routines, boundaries, and connections. Remember, taking care of your mental health isn’t a luxury—it’s essential for your productivity and success, both professionally and personally. Stay sharp, stay connected, and let's keep pushing the limits—not just surviving the remote work grind, but thriving in it.
What Sets Apart a Good Junior Developer from a Bad One?
Dan Tulpa -Let's cut to the chase. The difference between an outstanding junior developer and an average one can significantly impact the dynamics of your team. It greatly affects productivity and the success of your projects. Here's what sets apart the stars from the mediocre in the realm of budding developers.
Hunger for Learning
The Stars: The best junior developers are endlessly curious. They constantly seek knowledge, not limiting themselves to training manuals or tutorials. They delve into the codebase, experiment with new technologies, and learn by breaking and fixing things. Each code compile is a learning experience, whether it succeeds or fails.
The Mediocre: Then there are those who wait passively for knowledge. They avoid challenges, sticking to familiar ground and expecting to be guided at every step. This mindset stifles growth and drags the team down.
Problem-Solving Skills
The Stars: The developers you want on your team tackle problems head-on. They bring enthusiasm and fresh perspectives, unafraid to experiment or ask for help when needed. They approach issues with questions and evidence of their attempts to resolve them.
The Mediocre: On the other hand, some developers hit a minor obstacle and give up. They either offload the problem onto others or ignore it, hoping it resolves itself. Newsflash: it doesn’t.
Communication and Teamwork
The Stars: Effective communication goes beyond talking; it involves clearly exchanging ideas. Top junior devs articulate their thoughts, updates, and issues well. They listen attentively and appreciate feedback, knowing it’s crucial for their growth.
The Mediocre: Poor communicators act like black holes, rarely sharing or receiving information effectively. They isolate themselves and take feedback personally, making them poor team players.
Eye for the Details
The Stars: Attention to detail distinguishes a polished app from a buggy one. Exceptional developers meticulously review their code, catching bugs others might miss. They prioritize testing and review, understanding that quality is key.
The Mediocre: Rushed work and ticking off tasks without thorough review lead to sloppy results. This not only disrupts timelines but also adds unnecessary stress to the team.
Adaptability
The Stars: Technology evolves rapidly. The best developers adapt quickly, picking up new skills and tools as needed. They embrace change and are flexible with shifting priorities.
The Mediocre: Then there are those resistant to new methods or technologies, clinging to outdated practices. This reluctance hampers both project progress and personal growth.
Bottom Line
Being a standout developer goes beyond coding. It's about being a continuous learner, an effective communicator, a detail-oriented worker, and a flexible team player. These qualities transform someone into a valuable asset for any development team. Lacking these traits? Then you're more of a liability. As technology progresses, nurturing these characteristics early on can pave the way for a successful career in software development.
Job Hunting with AI: The No-Nonsense Guide to Ethical Use
Dan Tulpa -In the current cutthroat arena of tech job hunting, AI tools like ChatGPT have become absolute game changers. They’re reshaping how we craft resumes, personalize cover letters, and prep job interviews. But let's get real — relying on AI raises some SERIOUS ethical flags. This guide isn't just about leveraging AI; it’s about using it smartly and standing tall with integrity in a sea of competitors.
AI in Job Searches: A Double-Edged Sword
Sure, AI can turbocharge your job search by matching you with the right gigs and polishing up your application docs. But leaning too hard on AI? That could strip the soul out of your applications, leaving those who pour genuine effort into their resumes and cover letters at a disadvantage.
Keeping It Real: Ethical AI Practices
AI as Your Sidekick, Not Your Stand-In!
Think of AI as your ally, not your ghostwriter. It’s there to spot typos and finesse your phrasing, but the real substance—your experiences and wins—must be 100% you.
Personalize, Don’t Automate
AI’s cool for tailoring your docs to the job ad, but at least TRY to infuse them with your own voice. Show recruiters your genuine interest and how well you mesh with their culture.
Play Fair in Tech Assessments
Use AI to get a grip on programming concepts or pinpoint weak spots, but pass those coding tests on your own steam. Cheating with AI solutions not only tarnishes your rep but also undercuts the whole point of the screening process.
How to Rock AI in Your Job Search
Boost, Don't Replace, Personal Effort:
- Resume Tips: Let AI help you echo the job description’s language, but ensure it’s your achievements that shine through.
- Cover Letters: Start with an AI-assisted draft, but pack it with your personal motivations and insights.
- Coding Prep: Have AI propose practice problems or clarify coding concepts, but solve real challenges honestly to display your actual skills.
Maintain Integrity and Authenticity:
- Be upfront about your AI use, especially in interviews. Owning up to it shows you’re ethical and thoughtful about your methods.
- Question AI’s advice. It’s not all gold—pick what truly represents you.
Balance AI Help with Personal Development:
- Invest time in soft skills — communication, leadership, creativity — that AI can’t replicate but are key in quite literally every job.
- Keep learning and stay flexible. The job market and tech are always evolving, and so should you.
Conclusion
AI is a powerhouse tool for job seekers when used wisely and ethically, ramping up both efficiency and effectiveness in your hunt. But remember, the essence of your application—personal touch, original ideas, and hard work—is something AI can never replace. By utilizing AI tools responsibly, you’ll not only boost your job search but also ensure you’re respecting the process and your fellow candidates. Let AI supplement your skills, not substitute them.
Kicking Imposter Syndrome to the Curb: A Developer's Guide
Dan Tulpa -Feeling like a fraud who's just winging it in the tech world? Yeah... you're not alone. Imposter syndrome hits hard, especially in a field that moves as fast as tech. It doesn't matter if you're fresh out of the gate or you've been in the game for years; that nagging doubt that you're just one mistake away from being exposed can chew you up. But here’s the kicker:
Ready???
it’s all in your head!. This post is all about getting real with yourself, ditching those doubts, and owning your place in the tech world.
What’s Up With Imposter Syndrome?
Imposter syndrome is that annoying voice in your head that keeps telling you you're not good enough, that you’re going to get busted for not knowing enough. It’s not about actual skills or knowledge gaps—it’s all about self-doubt. And it’s a beast that can mess with anyone, at any stage of their career.
Tips for the Rookie Developer
Hit the Books, But Don’t Freak About Being Perfect: Learning is a marathon, not a sprint. Get comfortable with being uncomfortable and remember that every expert was once a beginner.
Get Your Backup: Look into communities like Stack Overflow or GitHub, or hit up local meetups. Realizing you’re not the only one struggling can be a massive relief.
Advice for the Mid-Level Developer
Track Your Wins: Keep a log of the kudos you’ve received, projects you’ve nailed, and the positive feedback that's come your way. When doubt creeps in, give that list a read.
Teach What You Know: There’s no better way to demolish self-doubt than by helping someone else learn the ropes. Plus, teaching cements your own knowledge like nothing else.
Cultivate Curiosity: Find a team vibe where it’s cool to ask questions and where continuous learning trumps know-it-all behavior. This sets everyone up for success, without the pressure cooker environment.
Universal Tactics to Crush Imposter Syndrome
Spit It Out!
Talking about imposter syndrome strips away its power. Odds are, many of your colleagues feel the same way. Shedding light on it means you can all tackle it together.
Learn, Don’t Compare
Tech’s too vast for anyone to know it all. Focus on your own growth path instead of measuring yourself against someone else’s highlight reel.
Embrace the Mess-Ups
Screwing up doesn’t make you a fake; it makes you human. Every mistake is a step towards mastery, so don’t beat yourself up.
Cheer Even the Small Victories
Got through a tough bug? Celebrate that win. Small victories stack up and help build resilience and a positive outlook.
Crave Feedback
Seek out constructive criticism. It’s not just about finding flaws - it’s about understanding where you shine and where you can polish up your skills.
Wrap-Up
Imposter syndrome is just part of the developer’s journey, but it doesn’t define you. It’s about how you handle the hurdles, keep learning, and value your unique journey in tech. Next time you feel like an imposter, remember: it’s not just you. You’ve got this, and you’re exactly where you need to be.